SoundManager
Manage sound features. The AudioSource required for playback is automatically generated and managed internally.
namespace GarageKit
public class SoundManager : ManagerBase
Inheritance
SoundManager
-> ManagerBase -> MonoBehaviour
Inspector
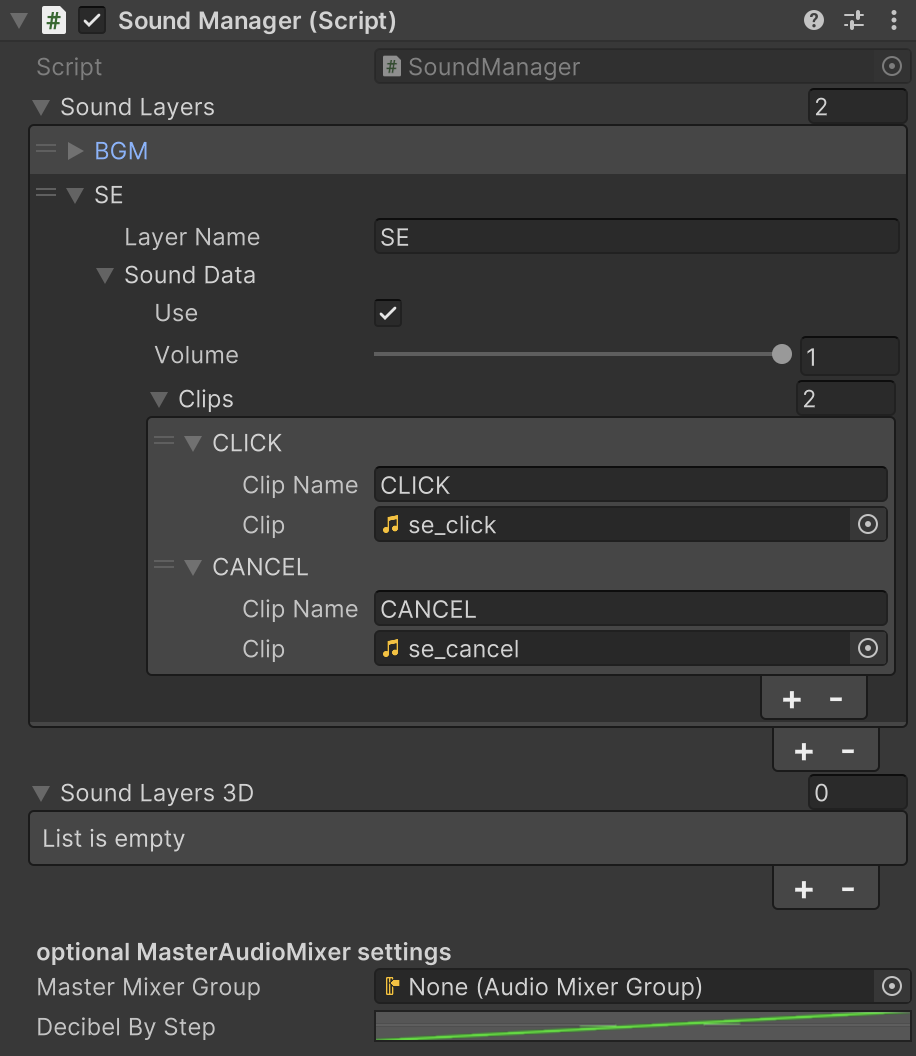
Properties
member | type | description |
---|---|---|
soundLayers | List<SoundLayerData> | Sound setting |
soundLayers3D | List<Sound3DLayerData> | 3D Sound setting |
SoundLayers | Dictionary<string, SoundLayerData> | Table with sound layer names |
SoundLayers3D | Dictionary<string, Sound3DLayerData> | Table with 3D sound layer names |
masterMixerGroup | AudioMixerGroup | Option: Master mixer group |
decibelByStep | AnimationCurve | Option: Master volume step adjustment curve |
Methods
Add sound layer
public void AddLayer(SoundLayerData layer)
Add AudioClip to sound layer
public void AddClip(string layerName, string clipName, AudioClip clip)
Sound playback
public void Play(string layerName, string clipName, bool overlap = false)
public void Play(string layerName, string clipName, bool overlap, bool loop, bool asOneShot)
Playback status of the sound layer
public bool IsPlay(string layerName)
Stop sound playback
public void Stop(string layerName = "")
Stop playing all sounds
public void StopAll(string layerName = "")
Add 3D sound layer
public void AddLayer3D(Sound3DLayerData layer)
3D 音声レイヤーに 3D 音声ソースの追加 Add 3D AudioSource to 3D sound layer
public void AddSource3D(string layerName, string sourceName, AudioSource source)
3D sound playback
public void Play3D(string layerName, string sourceName, bool overlap = false)
public void Play3D(string layerName, string sourceName, bool overlap, bool loop, bool asOneShot)
Playback status of the 3D sound layer
public bool IsPlay3D(string layerName, string sourceName)
Stop 3D sound playback
public void Stop3D(string layerName = "", string sourceName = "")
Stop playing all 3D sounds
public void Stop3DAll(string layerName = "")
Fade in all sounds
public void FadeInAllSound(float time = 1.0f)
Fade out all sounds
public void FadeOutAllSound(float time = 1.0f)
Fade of specified sound
public void Fade(string layerName, float fromVol, float toVol, float time)
Fade of specified 3D sound
public void Fade3D(string layerName, string sourceName, float fromVol, float toVol, float time)
Setting the master volume
public void SetMasterVol(float vol, string exposeProperty = "MasterVolume")
Master volume step up one
public void MasterVolUp(string exposeProperty = "MasterVolume")
Master volume step down one
public void MasterVolDown(string exposeProperty = "MasterVolume")
Example
// Normal playback
AppMain.Instance.soundManager.Play("SE", "CLICK");
// Loop playback without overlap
AppMain.Instance.soundManager.Play("BGM", "CLIP", false, true);
SoundClipData
Clip information structure
namespace GarageKit
[Serializable]
public class SoundClipData
Properties
member | type | description |
---|---|---|
clipName | string | Clip name |
clip | AudioClip | AudioClip reference |
SoundData
Sound information structure
namespace GarageKit
[Serializable]
public class SoundData
Properties
member | type | description |
---|---|---|
use | bool | Use it or not |
volume | float | Sound volume |
clips | List<SoundClipData> | Clip information list |
SoundLayerData
Layer information structure
namespace GarageKit
[Serializable]
public class SoundLayerData
Properties
member | type | description |
---|---|---|
layerName | string | Layer name |
soundData | SoundData | Sound information |
Methods
Disable processing for each layer
public bool IgnoreAllMethod { get; set; }
SoundSourceData
3D sound source information structure
namespace GarageKit
[Serializable]
public class SoundSourceData
Properties
member | type | description |
---|---|---|
sourceName | string | 3D sound source name |
source | AudioSource | AudioSource reference |
Sound3DData
3D sound information structure
namespace GarageKit
[Serializable]
public class Sound3DData
Properties
member | type | description |
---|---|---|
use | bool | Use it or not |
volume | float | SOund volume |
sources | List<SoundSourceData> | 3D sound source information list |
Sound3DLayerData
3D layer information structure
namespace GarageKit
[Serializable]
public class Sound3DLayerData
Properties
member | type | description |
---|---|---|
layerName | string | Layer name |
soundData | Sound3DData | Sound information |
Methods
Disable processing for each layer
public bool IgnoreAllMethod { get; set; }