SceneStateManager
Manage state transition functionality. Place and manage state objects that inherit StateBase under the SceneStateManager hierarchy.
namespace GarageKit
public class SceneStateManager : ManagerBase
Inheritance
SceneStateManager
-> ManagerBase -> MonoBehaviour
Inspector
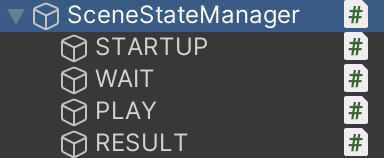
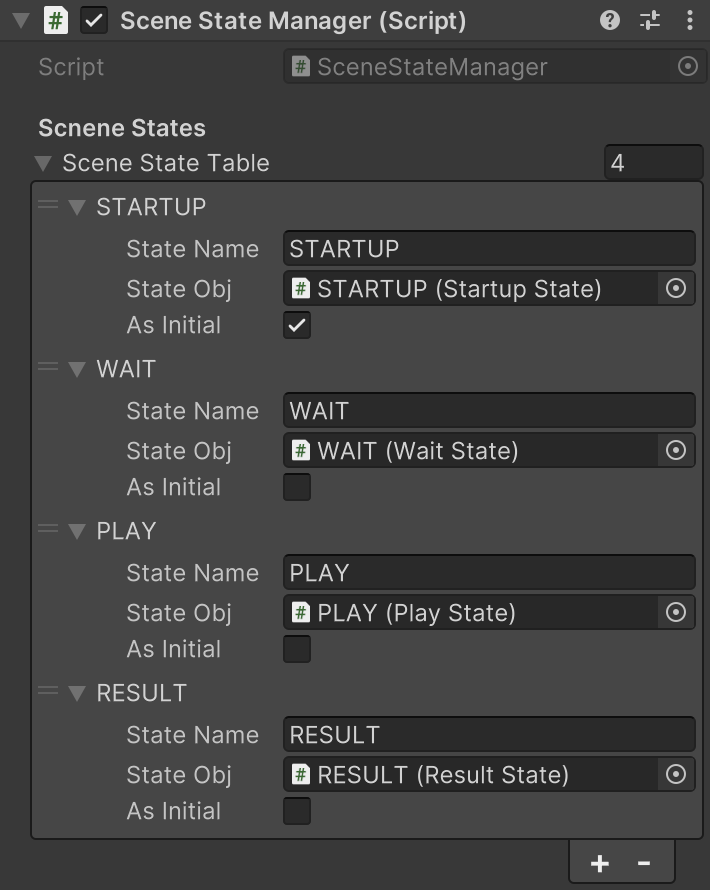
Properties
member | type | description |
---|---|---|
sceneStateTable | List<SceneStateData> | State transition information table |
Methods
Current state information
public SceneStateData CurrentState { get; }
Previous state name
public string FromStateName { get; }
State transition processing flag
public bool StateChanging { get; }
State transition fade processing flag
public bool AsyncChangeFading { get; }
State initialization completion flag
public bool StateInitted { get; }
Get state object from scene
public T FindStateObjectOfType<T>() where T : StateBase
public StateBase FindStateObjectByName(string stateName)
Transition to initial state. called WaitForEndOfFrame()
from Start()
in AppMain.
public void InitState()
Transition to specified state
public void ChangeState(string stateName, object context = null)
Transition with fade to specified state
public void ChangeAsyncState(string stateName, object context = null)
Example
// Transition to specified state
AppMain.Instance.sceneStateManager.ChangeState("PLAY");
// Transition with fade by passing parameters to specified state
AppMain.Instance.sceneStateManager.ChangeAsyncState("PLAY", "param");
SceneStateData
State transition information structure
namespace GarageKit
[Serializable]
public class SceneStateData
Properties
member | type | description |
---|---|---|
stateName | string | State name |
stateObj | StateBase | Reference to state object |
asInitial | bool | Initial state flag |